-
- garciaolais
- Posts: 2
- Joined:
Binding Code Behind
Greetings !
I want to modify a sample 3D Widget app.
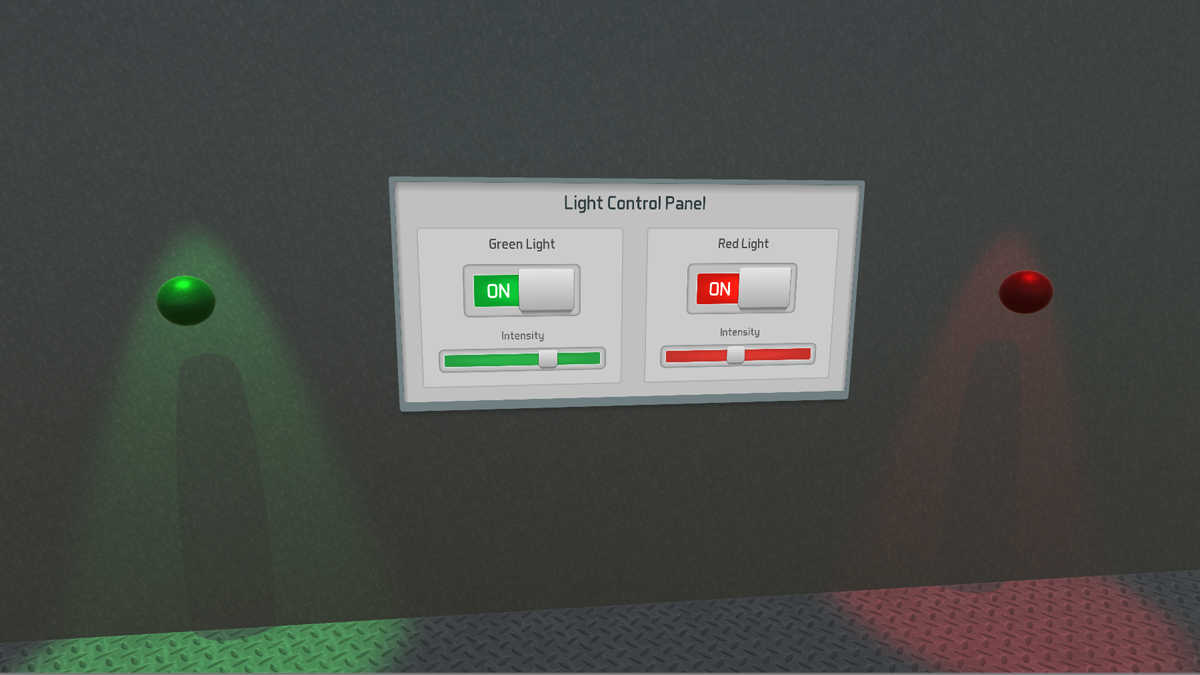
I want to display a switch value in the TextBlock. I've changed the xaml code and I add a binding but only update once.
I've changed the value "Lights Control Panel" for a {Binding redLight.enabled}
Thanks in advance!
I want to modify a sample 3D Widget app.
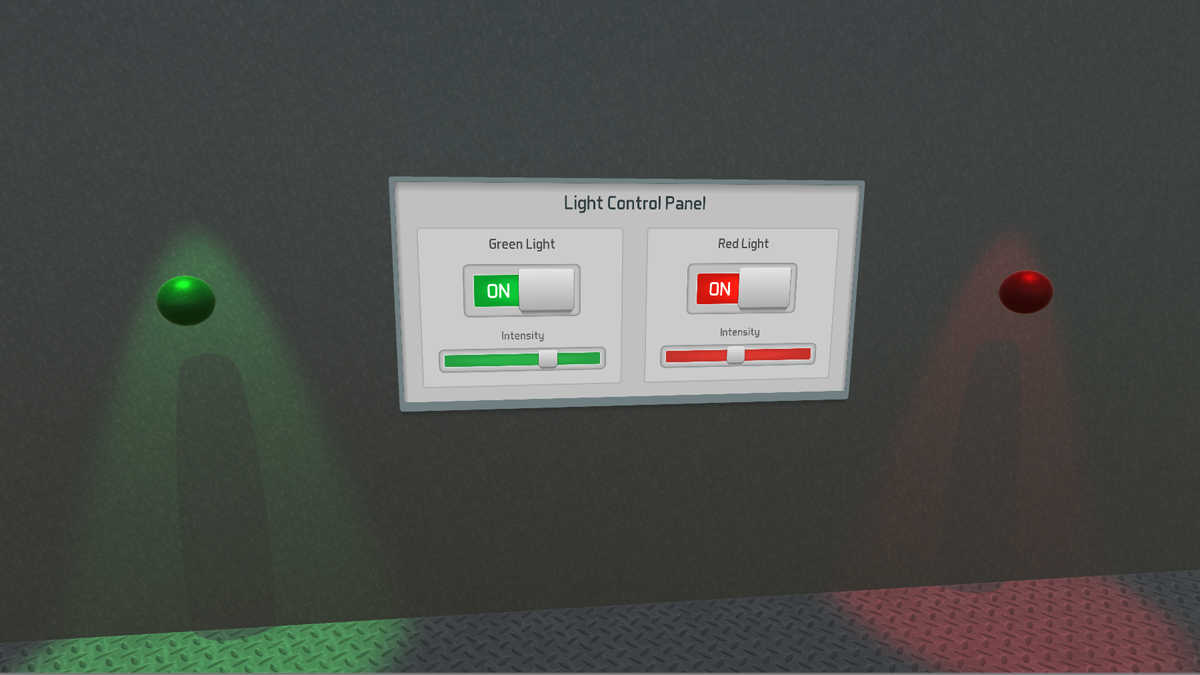
I want to display a switch value in the TextBlock. I've changed the xaml code and I add a binding but only update once.
I've changed the value "Lights Control Panel" for a {Binding redLight.enabled}
Code: Select all
<TextBlock Text="{Binding redLight.enabled}" HorizontalAlignment="Center" Grid.ColumnSpan="2" Margin="0,10,0,0" FontSize="16" VerticalAlignment="Top" Foreground="#FF324043"/>
-
-
sfernandez
Site Admin
- Posts: 3005
- Joined:
Re: Binding Code Behind
Hi,
First thing to explain is that you can only bind to public properties of the ViewModel (not fields), so you should use RedLightOn property instead.
The second thing to remark is that UI will only be updated if ViewModel changes are notified. To do that the ViewModel should implement the INotifyPropertyChanged interface and raise the PropertyChanged event with the name of the property being modified.
Please take a look at Data Binding tutorial to know more about this.
First thing to explain is that you can only bind to public properties of the ViewModel (not fields), so you should use RedLightOn property instead.
The second thing to remark is that UI will only be updated if ViewModel changes are notified. To do that the ViewModel should implement the INotifyPropertyChanged interface and raise the PropertyChanged event with the name of the property being modified.
Please take a look at Data Binding tutorial to know more about this.
-
- garciaolais
- Posts: 2
- Joined:
Re: Binding Code Behind
Hi
Thank you for your reply!!. I've read the documentation and I've made a change in my code and it works
ViewModel
xaml
.... and these is my little demo.
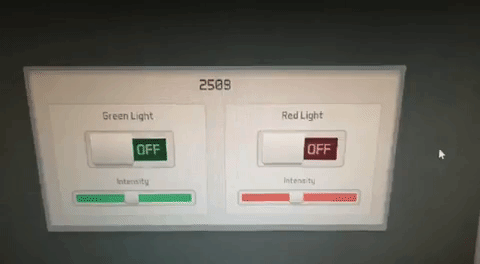
Thank you for your reply!!. I've read the documentation and I've made a change in my code and it works

ViewModel
Code: Select all
namespace Sample
{
public class TestBinding : INotifyPropertyChanged
{
private int _counter;
public int Counter
{
get { return _counter; }
set
{
if (_counter != value)
{
_counter = value;
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs("Counter"));
}
}
}
}
public event PropertyChangedEventHandler PropertyChanged;
}
public class ViewModel : MonoBehaviour
{
public Light redLight;
public Light greenLight;
public bool RedLightOn
{
get { return redLight.enabled; }
set { redLight.enabled = value; }
}
public float RedLightIntensity
{
get { return redLight.intensity; }
set { redLight.intensity = value; }
}
public bool GreenLightOn
{
get { return greenLight.enabled; }
set { greenLight.enabled = value; }
}
public float GreenLightIntensity
{
get { return greenLight.intensity; }
set { greenLight.intensity = value; }
}
private TestBinding _testBinding;
public TestBinding testBinding
{
get { return _testBinding; }
set
{
if (_testBinding != value)
{
_testBinding = value;
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs("testBinding"));
}
}
}
}
public event PropertyChangedEventHandler PropertyChanged;
private void Start()
{
NoesisView view = GetComponent<NoesisView>();
view.Content.DataContext = this;
_testBinding = new TestBinding();
}
private void Update()
{
_testBinding.Counter++;
Debug.Log(_testBinding);
}
}
}
xaml
Code: Select all
<TextBlock Text="{Binding testBinding.Counter}" HorizontalAlignment="Center" Grid.ColumnSpan="2" Margin="0,10,0,0" FontSize="16" VerticalAlignment="Top" Foreground="#FF324043"/>
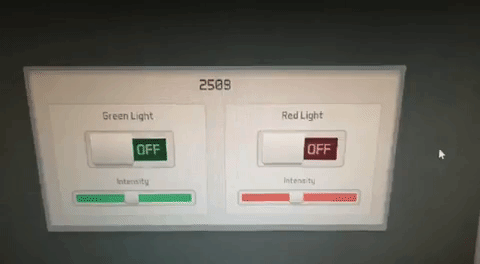
Re: Binding Code Behind
Great! Thank you, closing this
Who is online
Users browsing this forum: Ahrefs [Bot] and 6 guests